Structured, colourful, and configurable logging library
Transform your Node.js logging with @launchquest/log. Enjoy structured, colourful logs with customisable options, enhancing your debugging experience and making coding sessions more enjoyable and efficient.
Working on software can be dull. Or it can be exciting. We've all had days where the day has dragged, and coding feels like running through water, only to get home and... code, and the night disappears in an instant.
It's all about enjoyment and entering a state of flow. Part of that is enjoying the tools you work with. Many developers introduce frameworks into corporate environments that they first started using at home, which were most likely built by other developers at home. We call this grassroots innovation, where the tools we love in our personal projects become the tools we rely on professionally.
For me, I like colourful logs. Put it down to my first Linux experience being Gentoo, the colourful distro, during my formative years as a 12-year-old programmer. Logs don't have to be plain and boring; they can be vibrant and informative, making the debugging process more enjoyable and efficient.
I'm very particular about my logs, so I'd like to introduce my tiny yet powerful, and colourful, logging library: @launchquest/log
.
@launchquest/log
is a customisable logging library for Node.js, designed to provide a structured, colourful, and configurable logging experience. It supports multiple log levels and customisable options to fit various logging needs.
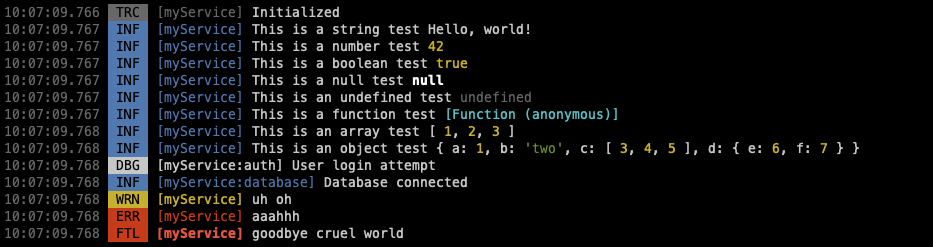
Installation
To install @launchquest/log
, run:
npm install @launchquest/log
Basic Usage
To use @launchquest/log
, first import it into your project:
import { Log } from '@launchquest/log'
const log = new Log()
log.info('This is an informational message')
log.warn('This is a warning message')
log.error('This is an error message')
Log Levels
@launchquest/log
supports six log levels, each with its own label and colour:
trace
: TRC (grey background)debug
: DBG (white background)info
: INF (blue background)warn
: WRN (yellow background)error
: ERR (red background)fatal
: FTL (red background, bold text, triggersprocess.exit
by default)
log.trace('This is a trace message')
log.debug('This is a debug message')
log.info('This is an informational message')
log.warn('This is a warning message')
log.error('This is an error message')
log.fatal('This is a fatal error message')
Customisation Options
When creating a new @launchquest/log
instance, you can customize various options:
name
: A name to prefix all log messages (default:''
)timestamp
: The timestamp format (default:'HH:mm:ss.SSS'
)logLevel
: The minimum log level to display (default:'info'
)exitOnFatal
: Whether to exit the process on a fatal error (default:true
)
const log = new Log({
name: 'myApp',
timestamp: 'YYYY-MM-DD HH:mm:ss',
logLevel: 'debug',
exitOnFatal: false
})
log.info('This is an informational message with custom settings')
Named Loggers
You can create named child loggers using the extend
method:
const log = new Log({ name: 'app' })
const dbLog = log.extend('database')
dbLog.info('Database connected')
log.info('App started')
Output:
09:44:32.010 INF [app:database] Database connected
09:44:32.010 INF [app] App started
Full Example
Here’s a complete example showcasing various features:
import { Log } from '@launchquest/log'
const log = new Log({
name: 'myService',
timestamp: 'HH:mm:ss.SSS',
logLevel: 'trace',
exitOnFatal: true
})
log.trace('Initialized')
log.info('This is a string test', 'Hello, world!')
log.info('This is a number test', 42)
log.info('This is a boolean test', true)
log.info('This is a null test', null)
log.info('This is an undefined test', undefined)
log.info('This is a function test', () => {})
log.info('This is an array test', [1, 2, 3])
log.info('This is an object test', { a: 1, b: 'two', c: [3, 4, 5], d: { e: 6, f: 7] })
const authLog = log.extend('auth')
authLog.debug('User login attempt')
const dbLog = log.extend('database')
dbLog.info('Database connected')
This will output:
10:07:09.766 TRC [myService] Initialized
10:07:09.767 INF [myService] This is a string test Hello, world!
10:07:09.767 INF [myService] This is a number test 42
10:07:09.767 INF [myService] This is a boolean test true
10:07:09.767 INF [myService] This is a null test null
10:07:09.767 INF [myService] This is an undefined test undefined
10:07:09.767 INF [myService] This is a function test [Function (anonymous)]
10:07:09.768 INF [myService] This is an array test [ 1, 2, 3 ]
10:07:09.768 INF [myService] This is an object test { a: 1, b: 'two', c: [ 3, 4, 5 ], d: { e: 6, f: 7 } }
10:07:09.768 DBG [myService:auth] User login attempt
10:07:09.768 INF [myService:database] Database connected
10:07:09.768 WRN [myService] uh oh
10:07:09.768 ERR [myService] aaahhh
10:07:09.768 FTL [myService] goodbye cruel world
Logging doesn't have to be a mundane task. With @launchquest/log
, you can make it structured, colourful, and enjoyable. Transform your logging experience and make your debugging sessions something to look forward to.